It’s time to make the world feel more alive. Just adding plants and trees won’t do the trick. Last time, we added soundscapes to the game, and it already feels a lot better, but we’re not there yet. Today, we’re going to add some life to our game by creating and animating various small creatures.
Modelling and Animation
Let’s fire up Blender and start with something simple: a butterfly. Two wings, a small head and tail, a shiny texture, and voila, our first little critter is ready to go. Job done, next task! Okay, that’s a blatant lie. There’s A LOT more to do.
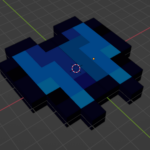
First of all, our butterfly needs to flap its wings. So, we import it to Unity, add a new animator, and spend some time creating a simple animation by rotating its wings along the forward axis. Getting the timing right and making the movement look natural takes some trial and error, but the results are promising.
While we’re at it, why not create more animals and practice modeling and texturing along the way? We could use some birds and fish. Naturally, they are more complex to model, but following our low-poly approach, they can be designed fairly quickly.
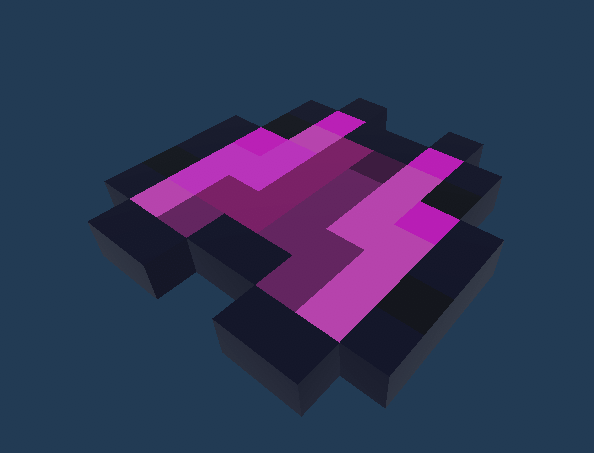
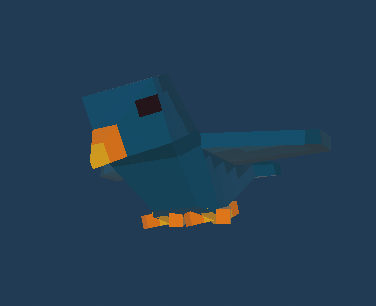
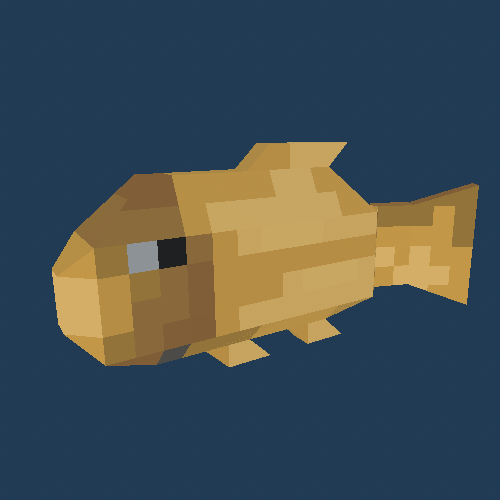
Behavior
We could now place these beautiful creatures in the scenes randomly or let them fly and swim around in straight lines. But I think we can do better. Let’s define their behavior on paper first and start with the easiest one.
Butterfly:
- Start attached to any surface and just idle around.
- If the player comes close, fly away.
- Change trajectory randomly at random intervals.
- If you hit another surface, attach to it and idle.
Okay, that sounds simple enough. Implementing that behavior and tweaking the parameters (movement speed, flap speed, trajectory changes, etc.) looks like this:
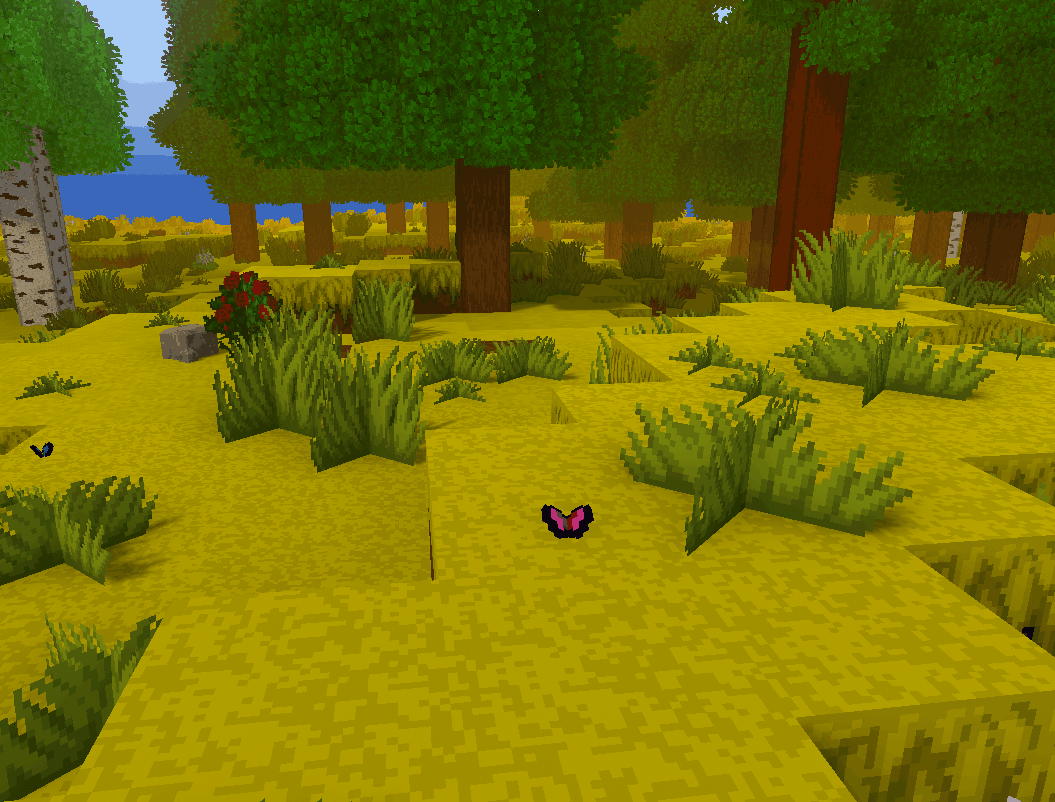
Birds are a bit more complex since they can’t grab onto all surfaces. Instead, we need to ensure that the surface the bird hits while flying is not too steep or upside down (we’ll leave ceiling-grabbing for the bats). Our updated rules:
Birds:
- Start attached to a flat, less than 50-degree angled surface (grass, top of a tree, roof) and just idle around.
- If the player comes close, fly away.
- Change trajectory randomly at random intervals.
- If you hit another surface, check if its angle is less than 50 degrees. If so, attach to it and idle. Otherwise, turn around and continue the flight.
And that logic implemented looks like this:
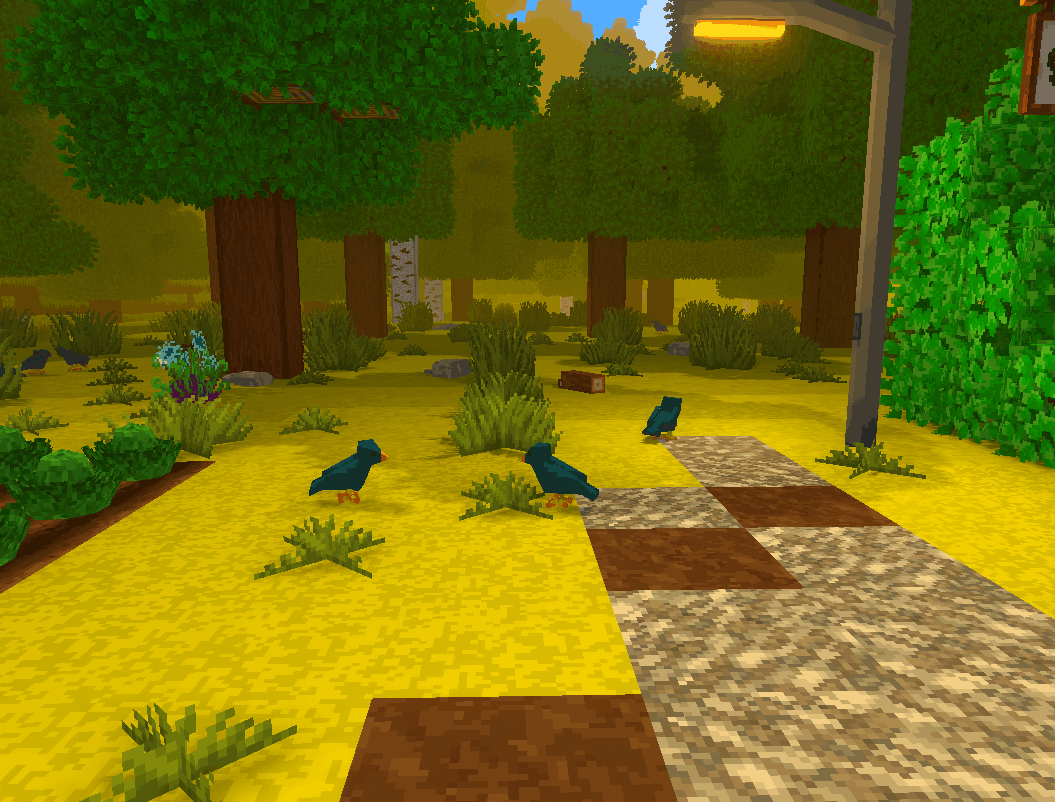
Fish, on the other hand, are a completely different beast. They don’t follow any of the behaviors stated above. Instead, they mostly swim and move around constantly, group into schools, and avoid obstacles and threats. Luckily for us, someone already thought of this in 1986, and I’m happy to use the ancient knowledge of Boids to make our fish behave fishy. So, what is Boids in the first place? Boids, or Bird-oid Objects, describes the behavior of flocks of birds or fish by applying simple rules to every actor in a group, which make the group stick together while avoiding obstacles and threats. These rules are:
Fish:
- Move forward constantly. If you see any obstacle in your path (wall, another fish), steer away from it.
- If there are any fish of your species close to you, average their speed and direction and steer towards this speed and direction yourself.
- If there are any fish of your species close to you, average their position and slightly steer towards this point.
Different to butterfly and bird rules, these behavior patterns are additive, so a fish will always apply all of them, if possible. It is about setting custom weights to each rule to make it work proper.
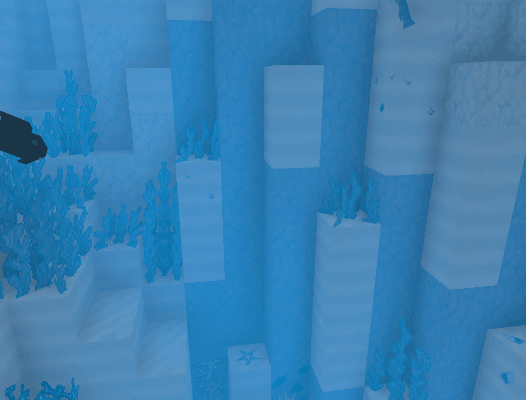
This requires a lot of tweaking with the different weights and how exactly to apply them. Even slight changes can make the group behave in almost completely different pattern, from extreme cohesion till almost permanent go-solo. Playing around with those mechanics showed, that in order to make it look really good, we need to add two extra rules:
- Add random noise to the current trajectory over time to make the fish’s movement more varied and prevent lonely fish from swimming in straight lines only.
- If the player comes close, steer away and accelerate for a short duration.
Yesss, be afraid of me!
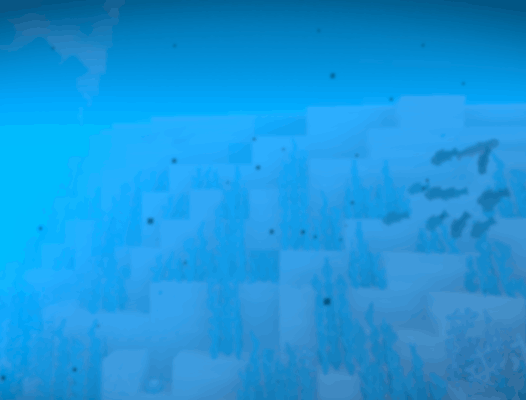
Spawning
Phew. We’ve talked a lot about behavior, but we haven’t decided how to spawn these animals. I think we’ll keep it super simple:
- Check every second for a spot to spawn a creature, at least 30 blocks away from the player.
- Spawn a creature (or a bunch) if possible (flat surface for birds, water for fish).
- Repeat until a maximum count of animals is spawned.
- If the player moves too far away from these animals, remove them.
Sure, there are more details to pay attention to when implementing the spawning algorithm, but the base rules are solid. So, after putting in so much work, what could possibly go wrong?
Oh no … water repellent fish, giant fish, cursed birds …
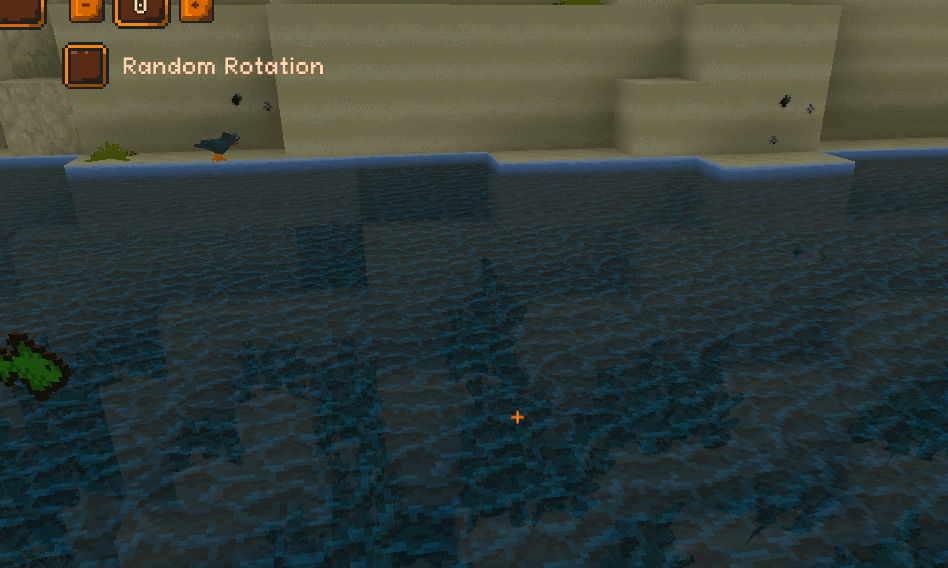
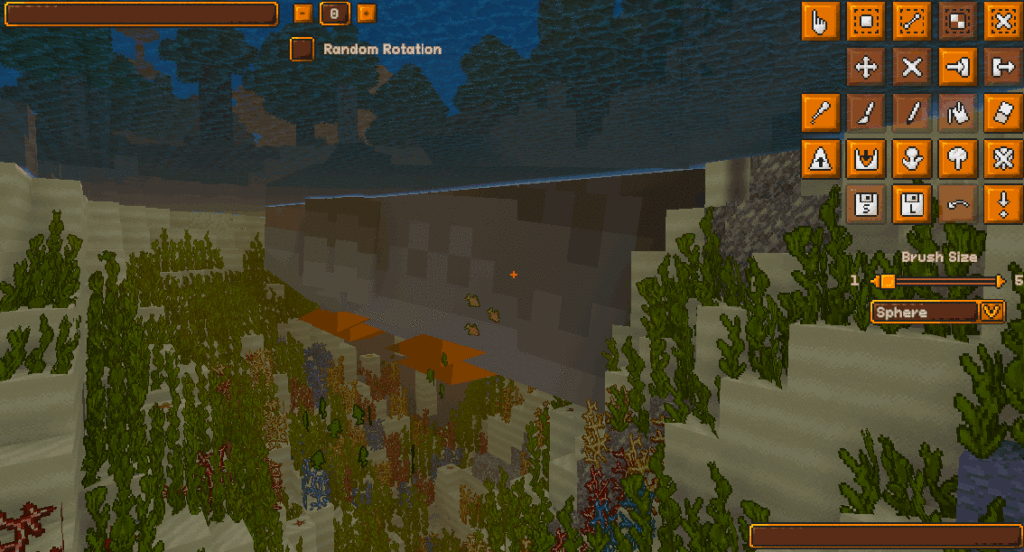
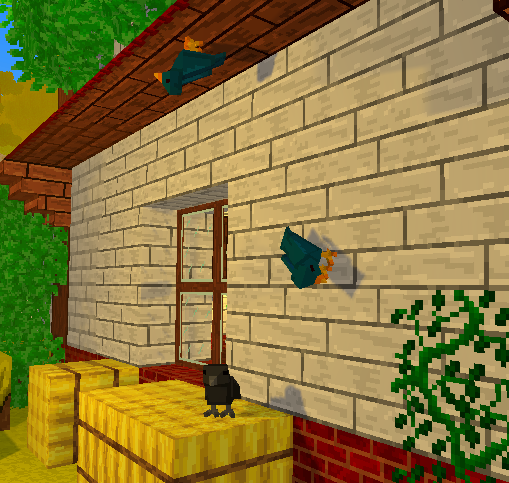
So, fixing those bugs, which was surprisingly fun, I added different kind of animals, including custom animations. Just a small teaser here, if you want to see all of them, you probably have to play the game yourself 🙂
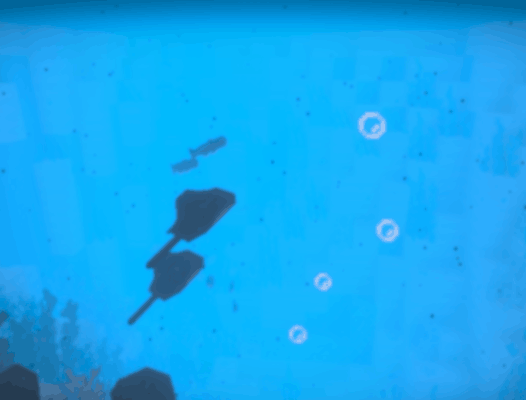
Leave a Reply